How Do You Loop the Same Thread Over and Over Again in Java
Chapter 6
Threads and Multithreading in Coffee
past Debasis Samanta
- Introduction
- Nuts of a thread
- Creating and Running a Thread
- Life cycle of threads
- Condition of a Thread
- Synchronization and Inter-Thread Advice
- Synchronization
- Inter-thread Advice
- Thread Groups and Daemon
- Do Questions
- Assignment
- Q&A
Introduction
Multi-threading means multiple flow of control. Multi-threading programming is a conceptual epitome for programming where 1 can split up a program into ii or more than processes which tin can be run in parallel. In that location are two master advantages of multi-threading : Fist, program with multiple threads will, in general, result in better utilization of organisation resources, including the CPU, because another line of execution can grab the CPU when i line of execution is blocked. Second, there are several problems better solved by multiple threads. For example, nosotros can easily write a multi-threaded program to testify animation, play music, display documents, and downwardly load files from the network at the aforementioned time.
Coffee is a multi-threaded language. Java allows to write a program where more than one processes tin be executed concurrently within the single program. Coffee's threads are often referred to every bit light weight threads, which means that they run in the aforementioned memory infinite. Because Coffee threads run in the same retentivity space, they tin can hands communicate among themselves considering an object in 1 thread tin can call a method in some other thread without whatever overhead from the operating system. In this Tutorial we will learn how to do multi-threaded programming in Java.
Basics of a thread
As with the Java concepts, everything about thread are defined in a class Thread. The Thread grade encapsulates all of the command 1 volition need over threads. The Thread form is our only link to manage how threads comport. In this Section, we will acquire nigh : how to create and run a thread, the life cycle of a thread, and the thread controlling methods.
Creating and Running a Thread
There are ii ways to define a thread: i is sub classing Thread and other is using the Runnable interface.
Using the sub classing thread : With this method, we have to define a class as a sub class of the Thread class. This sub course should contain a body which volition exist divers by a method run(). This run() method contains the bodily job that the thread should perform. An example of this sub class is then to be created past a new statement, followed by a call to the thread's start() method to have the run() method executed. Allow united states of america consider the Illustration half dozen.1 which includes a plan to create at that place individual threads that each print out their own " Hello Earth !" string.
Analogy six.one // Creating and running threads using sub classing Thread // /* Creating three threads using the class Thread and and so running them concurrently. */ class ThreadA extends Thread{ public void run( ) { for(int i = one; i <= five; i++) { Organisation.out.println("From Thread A with i = "+ -i*i); } System.out.println("Exiting from Thread A ..."); } } class ThreadB extends Thread { public void run( ) { for(int j = 1; j <= v; j++) { Arrangement.out.println("From Thread B with j= "+2* j); } System.out.println("Exiting from Thread B ..."); } } class ThreadC extends Thread{ public void run( ) { for(int k = i; m <= 5; k++) { System.out.println("From Thread C with one thousand = "+ (2*one thousand-i)); } System.out.println("Exiting from Thread C ..."); } } public course Demonstration_111 { public static void primary(String args[]) { ThreadA a = new ThreadA(); ThreadB b = new ThreadB(); ThreadC c = new ThreadC(); a.get-go(); b.first(); c.outset(); System.out.println("... Multithreading is over "); } }
OUTPUT: |
From Thread A with i = -1 From Thread A with i = -two From Thread A with i = -3 From Thread B with j= ii From Thread A with i = -4 From Thread A with i = -v Exiting from Thread A ... ... Multithreading is over From Thread C with thou = 1 From Thread B with j= 4 From Thread B with j= 6 From Thread B with j= viii From Thread B with j= x Exiting from Thread B ... From Thread C with m = 3 From Thread C with one thousand = 5 From Thread C with 1000 = seven From Thread C with k = 9 Exiting from Thread C ... |
In the above simple example, three threads (all of them are of some type) volition be executed concurrently. Note that a thread tin can be directed to start its body by start() method.
Using the Runnable interface : A 2nd way to create threads is to make use of the Runnable interface. With this approach, first we take to implement the Runnable interface.[Runnable interface is already defined in the system bundle coffee.lang with a single method run() every bit below :
public interface Runnable { public abstract void run( ); } ]
When nosotros volition create a new thread, actually a new object will be instantiated from this Runnable interface every bit the target of our thread, meaning that the thread will look for the code for the run( ) method within our object's class instead of inside the Thread's class. This is illustrated with an instance where two processes Brother and Sis will be executed simultaneously.
Illustration 6.ii /* Creating iii threads using the Runnable interface and and so running them concurrently. */ class ThreadX implements Runnable{ public void run( ) { for(int i = 1; i <= 5; i++) { System.out.println("Thread X with i = "+ -1*i); } Organization.out.println("Exiting Thread X ..."); } } class ThreadY implements Runnable { public void run( ) { for(int j = i; j <= 5; j++) { System.out.println("Thread Y with j = "+ ii*j); } System.out.println("Exiting Thread Y ..."); } } class ThreadZ implements Runnable{ public void run( ) { for(int k = ane; grand <= 5; one thousand++) { Organisation.out.println("Thread Z with k = "+ (2*grand-1)); } Organization.out.println("Exiting Thread Z ..."); } } public course Demonstration_112 { public static void master(String args[]) { ThreadX 10 = new ThreadX(); Thread t1 = new Thread(x); ThreadY y = new ThreadY(); Thread t2 = new Thread(y); //ThreadZ z = new ThreadZ(); //Thread t3 = new Thread(z); Thread t3 = new Thread(new ThreadZ()); t1.start(); t2.offset(); t3.start(); Arrangement.out.println("... Multithreading is over "); } }
OUTPUT: |
Thread X with i = -ane Thread Ten with i = -two Thread Z with k = one Thread Z with k = 3 Thread Z with k = 5 Thread Z with grand = vii Thread Z with grand = 9 Exiting Thread Z ... ... Multithreading is over Thread Y with j = 2 Thread Y with j = four Thread Y with j = 6 Thread Y with j = eight Thread Y with j = ten Exiting Thread Y ... Thread X with i = -3 Thread X with i = -4 Thread 10 with i = -five Exiting Thread X ... |
Note : Annotation in the above case, how after implementing objects, their thread is created and their threads start execution. Too note that, a class instance with the run( ) method defined inside must be passed in equally an argument in creating the thread example so that when the start() method of this Thread instance is called, Java run time knows which run() method to execute. This alternative method of creating a thread is useful when the grade defining run() method needs to be a sub class of other classes; the class can inherit all the information and methods of the super class.
Life cycle of threads
Each thread is always in one of 5 states, which is depicted in Figure six.i
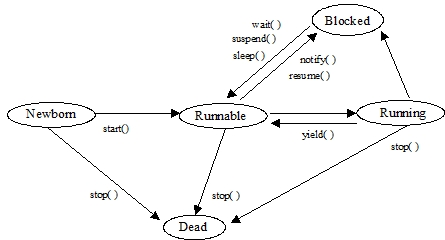
Newborn : When a thread is created (by new statement ) but not yet to run, information technology is called in Newborn country. In this state, the local data members are allocated and initialized.
Runnable : The Runnable land means that a thread is ready to run and is pending for the control of the processor, or in other words, threads are in this country in a queue and look their turns to be executed.
Running : Running means that the thread has control of the processor, its code is currently beingness executed and thread will continue in this country until it get preempted by a higher priority thread, or until it relinquishes command.
Blocked : A thread is Blocked means that it is being prevented from the Runnable ( or Running) country and is waiting for some event in order for it to reenter the scheduling queue.
Dead : A thread is Dead when information technology finishes its execution or is stopped (killed) by another thread.
Threads move from one state to another via a multifariousness of means. The common methods for decision-making a thread'due south land is shown in Figure vi.1. Below, nosotros are to summarize these methods :
start ( ) : A newborn thread with this method enter into Runnable state and Coffee run time create a system thread context and starts it running. This method for a thread object tin be called in one case simply
end( ) : This method causes a thread to end immediately. This is often an abrupt way to stop a thread.
append( ) : This method is different from stop( ) method. It takes the thread and causes information technology to stop running and later on tin can be restored past calling it again.
resume ( ) : This method is used to revive a suspended thread. At that place is no gurantee that the thread will start running right mode, since there might be a higher priority thread running already, merely, resume()causes the thread to become eligible for running.
sleep (int northward ) : This method causes the run time to put the electric current thread to sleep for n milliseconds. After n milliseconds have expired, this thread will go elligible to run once more.
yield( ) : The yield() method causes the run time to switch the context from the current thread to the next available runnable thread. This is i way to ensure that the threads at lower priority do not get started.
Other methods like await(), notify(), join() etc. will be discussed in subsequent discussion. Let us illustrate the use of these method in a elementary Application.
Analogy 6.3 /* Demonstration of thread grade methods : getID() */ /* Java code for thread creation past extending the Thread class */ course ThreadId extends Thread { public void run() { endeavour { // Displaying the thread that is running System.out.println ("Thread " + Thread.currentThread().getId() + " is running"); } catch (Exception due east) { // Throwing an exception Organization.out.println ("Exception is caught"); } } } public class Demonstration_113{ public static void chief(Cord[] args) { int n = 8; // Number of threads for (int i=0; i<viii; i++) { ThreadId object = new ThreadId(); object.start(); } } }
OUTPUT: |
Thread 21 is running |
Illustration half-dozen.4 /* Demonstration of thread class methods : getID() */ /* Coffee code for thread cosmos by implementing the Runnable Interface */ class ThreadId implements Runnable { public void run() { attempt { // Displaying the thread that is running System.out.println ("Thread " + Thread.currentThread().getId() + " is running"); } catch (Exception east) { // Throwing an exception Organisation.out.println ("Exception is caught"); } } } // Primary Grade grade Demonstration_114 { public static void main(Cord[] args) { int n = viii; // Number of threads for (int i=0; i<8; i++){ Thread object = new Thread(new ThreadId()); object.start(); } } }
OUTPUT: |
Thread 21 is running |
Illustration half dozen.5 /* Use of yield(), stop() and sleep() methods */ grade ClassA extends Thread{ public void run() { Organization.out.println("Start Thread A ...."); for(int i = 1; i <= 5; i++) { if (i==i) yield(); Organization.out.println("From Thread A: i = "+ i); } Organisation.out.println("... Get out Thread A"); } } form ClassB extends Thread{ public void run() { System.out.println("Start Thread B ...."); for(int j = 1; j <= 5; j++) { System.out.println("From Thread B: j = "+ j); if (j==2) cease(); } System.out.println("... Exit Thread B"); } } class ClassC extends Thread{ public void run() { System.out.println("Outset Thread C ...."); for(int k = ane; k <= v; k++) { System.out.println("From Thread B: j = "+ k); if (1000==iii){ try{ sleep(1000); }catch(Exception e){} } } Organisation.out.println("... Go out Thread C"); } } public class Demonstration_115{ public static void chief (String args[]) { ClassA t1 = new ClassA(); ClassB t2 = new ClassB(); ClassC t3 = new ClassC(); t1.showtime(); t2.start(); t3.start(); System.out.println("... End of executuion "); } }
OUTPUT: |
Start Thread A .... Start Thread C .... Kickoff Thread B .... ... End of executuion From Thread A: i = 1 From Thread B: j = i From Thread B: j = 2 From Thread B: j = 1 From Thread A: i = ii From Thread A: i = 3 From Thread A: i = iv From Thread A: i = 5 ... Exit Thread A From Thread B: j = ii From Thread B: j = 3 From Thread B: j = 4 From Thread B: j = v ... Exit Thread C |
Illustration 6.6 /* Use of append() and resume() methods */ course Thread1 extends Thread { public void run( ) { endeavor{ System.out.println (" Outset thread starts running" ); sleep(10000); System.out.println (" Get-go thread finishes running" ); } grab(Exception eastward){ } } } course Thread2 extends Thread { public void run( ) { try{ Organization.out.println ( "Second thread starts running"); System.out.println ( "Second thread is suspended itself "); suspend( ); System.out.println (" Second thread runs again" ); } catch(Exception e){ } } } class Demonstration_116{ public static void main (String args[ ] ){ try{ Thread1 first = new Thread1( ); // Information technology is a newborn thread i.e. in Newborn state Thread2 2d= new Thread2( ); // another new born thread first.start( ); // first is scheduled for running second.start( ); // second is scheduled for running System.out.println("Revive the 2d thread" ); // If it is suspended second.resume( ); Organisation.out.println ("2nd thread went for 10 seconds slumber " ); second.sleep (10000); System.out.println ("Wake upwards second thread and finishes running" ); System.out.println ( " Demonstration is finished "); } grab(Exception east){ } } }
OUTPUT: |
Revive the second thread |
Status of a Thread
It is some fourth dimension essential to know some information about threads. There are number of methods defined in Thread which can be chosen for getting information about threads. Some of the nigh normally used methods for thread'due south condition are listed here :
currentThread( ) : The CurrentThread() is a static method returns the Thread object which is the currently running thread.
setName( String s) : The SetName() method is to assign a name s for a thread prior its execution. This, therefore, identifies the thread with a string name. This is helpful for debugging multi-threaded programs.
getName( ) : This method returns the current cord value of the thread'due south name equally set by SetName().
setPriority (int p) : This method sets the thread's priotity to an integer value p passed in. There are several predefined priotiry constants defined in class Thread : MIN-PRIORITY, NORM-PRIORTY and MAX-PRIORITY, which are ane, 5, and 10 respectively.
getPriority ( ) : This method returns the thread's current priority, a value between 1 and 10.
isAlive ( ) : This method returns true if the thread is started but not dead yet.
isDaemon ( ) : This method returns truthful if the thread is a daemon thread.
Following is the Illustration six.vii to give an idea how the above mentioned method may be utilized.
Illustration vi.7 // Status data of threads // /* Setting priority to threads */ class ClassA extends Thread{ public void run() { System.out.println("Commencement Thread A ...."); for(int i = 1; i <= 5; i++) { Organisation.out.println("From Thread A: i = "+ i); } Arrangement.out.println("... Exit Thread A"); } } class ClassB extends Thread{ public void run() { System.out.println("Start Thread B ...."); for(int j = 1; j <= 5; j++) { System.out.println("From Thread B: j = "+ j); } Organisation.out.println("... Go out Thread B"); } } class ClassC extends Thread{ public void run() { System.out.println("Start Thread C ...."); for(int k = ane; thousand <= v; k++) { System.out.println("From Thread B: j = "+ k); } Organization.out.println("... Get out Thread C"); } } form Demonstration_117{ public static void principal (String args[]) { ThreadA t1 = new ThreadA(); ThreadB t2 = new ThreadB(); ThreadC t3 = new ThreadC(); t3.setPriority(Thread.MAX_PRIORITY); t2.setPriority(t2.getPriority() + 1); t1.setPriority(Thread.MIN_PRIORITY); t1.start(); t2.kickoff(); t3.outset(); System.out.println("... Finish of executuion "); } }
OUTPUT: |
Showtime Thread A .... From Thread A: i = one From Thread A: i = ii From Thread A: i = 3 From Thread A: i = 4 From Thread A: i = five ... Exit Thread A ... End of executuion Start Thread B .... Start Thread C .... From Thread B: j = 1 From Thread B: j = 2 From Thread B: j = iii From Thread B: j = four From Thread B: j = v ... Get out Thread B From Thread B: j = 1 From Thread B: j = two From Thread B: j = iii From Thread B: j = iv From Thread B: j = 5 ... Exit Thread C |
Illustration half-dozen.8 /* Information race case. */ public form Demonstration_118 extends Thread { public static int x; public void run() { for (int i = 0; i < 100; i++) { x = x + one; ten = x - 1; } } public static void main(Cord[] args) { x = 0; for (int i = 0; i < thou; i++){ new Demonstration_118().start(); System.out.println(x); // x non always 0! } } }
Synchronization and Inter-Thread Advice
It is already mentioned that threads in Java are running in the aforementioned retention space, and hence it is like shooting fish in a barrel to communicate betwixt two threads. Inter-thread communications allow threads to talk to or wait on each other. Once again, because all the threads in a program share the same memory space, information technology is possible for two threads to access the same variables and methods in object. Problems may occur when 2 or more threads are accessing the same data meantime, for example, one thread stores data into the shared object and the other threads reads data, at that place can be synchronization trouble if the first thread has not finished storing the data before the second one goes to read it. So we need to take care to admission the information by simply ane thread process at a time. Java provides unique language level support for such synchronization. in this Department we will learn how synchronization mechanism and inter-thread communications are possible in Java.
Synchronization
To solve the critical section problem, one usual concept is known what is chosen monitor. A monitor is an object which is used as a mutually exclusive lock ( called mutex). But ane thread may own a monitor at a given fourth dimension. When a thread acquires a lock information technology is said to take entered the monitor. All other threads attempting to enter the locked monitor volition exist suspended until the owner thread exits the monitor. But in Java, there is no such monitor. In fact, all Java object have their ain implicit monitor associated with them. Here, the key word synchronized is used past which method (due south) or block of statements can be made protected from the simultaneous access. With this, when a class is designed with threads in mind, the class designer decides which methods should non be allowed to execute concurrently. when a class with synchronized method is instantiated, the new object is given its own implicit monitor. The entire time that a thread is inside of a synchronized method, all other threads that try to call any other synchronized method on the aforementioned instance accept to wait. In guild to leave the monitor and relinquish command of the object to the next waiting thread the monitor owner simply needs to return from the method.
Let united states illustrate this mechanism with a simple instance.
Suppose, we want to maintain a bank account of customers. Several transactions, such every bit deposite some amount to an business relationship and withdraw some amount from an account etc. are possible. Now, for a given account, if two or more transactions come simultaneously then only one transaction should exist immune at a fourth dimension instead of simulataneous transaction processing so that data inconsistency will never occur. So, what nosotros need is to synchronize the transaction. Illustration 6.9 is to implement such a task.
Illustration half-dozen.9 /* The following Coffee application shows how the transactions in a bank can be carried out concurrently. */ class Account { public int residuum; public int accountNo; void displayBalance() { System.out.println("Account No:" + accountNo + "Balance: " + balance); } synchronized void deposit(int amount){ balance = remainder + amount; System.out.println( amount + " is deposited"); displayBalance(); } synchronized void withdraw(int amount){ remainder = balance - corporeality; System.out.println( amount + " is withdrawn"); displayBalance(); } } class TransactionDeposit implements Runnable{ int amount; Account accountX; TransactionDeposit(Account 10, int amount){ accountX = x; this.amount = corporeality; new Thread(this).starting time(); } public void run(){ accountX.deposit(amount); } } grade TransactionWithdraw implements Runnable{ int corporeality; Account accountY; TransactionWithdraw(Account y, int corporeality) { accountY = y; this.amount = amount; new Thread(this).beginning(); } public void run(){ accountY.withdraw(amount); } } class Demonstration_119{ public static void main(Cord args[]) { Account ABC = new Account(); ABC.residue = one thousand; ABC.accountNo = 111; TransactionDeposit t1; TransactionWithdraw t2; t1 = new TransactionDeposit(ABC, 500); t2 = new TransactionWithdraw(ABC,900); } }
OUTPUT: |
500 is deposited Account No:111Balance: 1500 900 is withdrawn Business relationship No:111Balance: 600 |
In the above example, the keyword synchronized is used for the methods void deposite(..) and void withdraw(�) so that these two methods will never run for the same object case simultaneously.
Alternatively, if one wants to design a class that was not designed for multi-thread access and thus has non-synchronized methods, then it can be wrapped the call to the methods in a synchronized block. Here is the general grade of the synchronized statement :
synchronized (Object ) { block of statement(s) }where Object is whatsoever object reference. For example, make all the methods in Account form as non-synchronized (remove the synchronized key word). Then modify the code for run( ) in class TransactionDeposite and course TransactionWithdraw are as under :
public void run( ) { // in TransactionDeposite synchronized (accountX ) { accountX.deposite(corporeality ); } } public void run( ) { // in TransactionWithdraw synchronized (accountY ) { accountY.withdraw(amount ); } }
Y'all will get the same output.
Interested reader may try to run the program without using synchronization and detect the effect.
Note : In a grade, non-synchronized methods are meantime executable.
Inter-thread Communication
In that location are three ways for threads to communicate with each other. The first is through commonly shared data. All the threads in the same program share the same memory space. If an object is accessible to various threads and so these threads share access to that object's data member and thus communicate each other.
The 2d mode for threads to communicate is past using thread command methods. There are such three methods by which threads communicate for each other :
suspend ( ): A thread tin can suspend itself and wait till other thread resume it.
resume ( ): A thread can wake up other waiting thread (which is waiting using suspend() ) through its resume() method and then can run concurrently.
join ( ) :This method can be used for the caller thread to expect for the completion of called thread.
The third fashion for threads to communicate is the use of three methods; wait(), notify(), and notifyAll(); these are defined in class Object of package coffee.lang. Actually these three methods provide an elegant inter-process communication machinery to take care the deadlock situation in Java. As in that location is multi-threading in programme, deadlock may occur when a thread belongings the key to monitor is suspended or waiting for some other thread'southward completion. If the other thread is waiting for needs to get into the same monitor, both threads volition be waiting for ever. The uses of these three methods are briefed as below :
look ( ):This method tells the calling thread to surrender the monitor and make the calling thread expect until either a fourth dimension out occurs or some other thread telephone call the same thread's notify() or notifyAll() method.
Notify ( ): This method wakes upwardly the only one (first) waiting thread chosen wait() on the aforementioned object.
notifyAll( ): This method volition wake upward all the threads that have been called wait( ) on the same object.
At present, let us demonstrate the classical use of these methods. Illustration half-dozen.6 is for this purpose.
Illustration 6.10 : // Inter thread communication : Producer & Consumer trouble // class Q { // Q is a course containing two parallel processes int northward; boolean flag = fake; //PRODUCER synchronized void put( int north) { // Produce a value if(flag) { // Entry try wait( ); take hold of(InterruptedException eastward); // to the } // disquisitional section this.n = n; Organization.out.println( "Produce :" + n); // Critical Section flag = truthful; // Exit from the notify( ); // disquisitional section } //CONSUMER synchronized int go( ) { // Consume a value if(! flag) { // Entry try wait( ); catch(InterruptedException e); // to the } // disquisitional section System.out.println( "Consume :" + north); // Disquisitional Section flag = simulated; // Exit from the notify( ); // critical // section return( north ); } class Producer implement Runnable { // Thread for Producer process Q q; Producer ( Q q ) { // constructor this.q =q; new thread (this).get-go ( ) ; // Producer procedure is started } public void run( ) { // infinite running thread for Producer int i = 0; while (truthful ) q.put ( i++ ); } } class Consumer implement Runnable { // Thread for consumer process Q q; Consumer (Q q ) { // Constructor this.q = q; new Thread (this).start ( ); } public void run( ) { // infinite running thread for Consumer while (true) q.become ( ); } } course PandC { public static void master( String args[ ] ) { Q q = new Q( ); // an instance of parallel processes is created new Producer(q) ; // Run the thread for producer new Consumer (q); // Run consumer thread } }
To empathise this critical section problem in operating system design, user may exist referred to : Operating organization concept by Peterson and Sylberchotze , Addiction Wesley Inc.
Note : All three methods i.due east. wait(), notify(), and notifyAll() must only exist called from the within of synchronized methods.
Thread Groups and Daemon
There are 2 other variation in thread class known : ThreadGroup and Daemon. ThreadGroup, as its name implies, is a group of threads. A thread group tin can take both threads and other thread groups as its member elements. In Java, there is a default thread grouping called SystemThreadGroup, which is nothing only the Java run time itself. When a Java application is started, the Coffee run time creates the main thread group as a member of the system thread group. A main thread is created in the master thread grouping to run the chief( ) method of the Application. In fact, every thread instance is member of exactly one thread group. Past default, all new user created threads and thread groups) will become the members of the principal thread group. All the threads and thread in an application course a tree with the system thread group every bit the root.
Daemon threads are service threads. They exist to provide service threads. They exist to provide services to other threads. They usually run in an space loop and attending the client threads requesting services. When no other threads exist daemon thread is automatically ceased to exist.
A new thread group can be created by instantiating the thread group form. For example,
Threadgroup TG = new ThreadGroup ( ) ;
Thread T = new Thread ( TG) ;
These two statements creates a new thread group TG which contains a thread T as the only member.
To create a daemon thread, at that place is a method setDaemon() can be called simply after the creation of a thread and before the execution is started. For case, following two statement is to make a thread as demon thread.
Thread T = new Thread ( ) ;The constructor of the thread is a good candidate for making this method telephone call, Too, past default, all the threads created by a daemon thread are as well daemon thread.
T setDaemon (true);
Some commonly used methods for treatment thread groups and daemon are listed below :
getName ( ) :Returns the proper noun of the thread group .
setName ( ) :Sets the proper name of the thread group .
geParent ( ) :Returns the parent thread group of the thread group .
getMaxPriority :Returns the current maximum priority of the thread grouping.
activeCount ( ) :Returns the number of active threads in the thread group.
activeGroupCount :Returns the number of active threads groups in the thread group.
isDaemon ( ) :Returns true if the thread is a daemon thread.
setDaemon ( ) : Set the thread equally a daemon thread prior its starting execution.
Practice Questions
Practice 6.1 /* Practice of a multithreaded program using subclassing Thread */ class ThreadA extends Thread{ public void run( ) { for(int i = ane; i <= v; i++) { System.out.println("From Thread A with i = "+ -1*i); } System.out.println("Exiting from Thread A ..."); } } course ThreadB extends Thread{ public void run( ) { for(int j = 1; j <= five; j++) { Arrangement.out.println("From Thread B with j = "+ 2*j); } System.out.println("Exiting from Thread B ..."); } } form ThreadC extends Thread{ public void run( ) { for(int thou = 1; k <= 5; k++) { Organization.out.println("From Thread C with grand = "+ (ii*m-1)); } System.out.println("Exiting from Thread C ..."); } } class MultiThreadClass{ public static void main(String args[]) { ThreadA a = new ThreadA(); ThreadB b = new ThreadB(); ThreadC c = new ThreadC(); a.start(); b.start(); c.start(); System.out.println("... Multithreading is over "); } }
Practice 6.2 /* Practice of a multithreaded program using Runnable interface */ class ThreadX implements Runnable{ public void run( ) { for(int i = 1; i <= 5; i++) { System.out.println("Thread Ten with i = "+ i); } System.out.println("Exiting Thread 10 ..."); } } class ThreadY implements Runnable{ public void run( ) { for(int j = 1; j <= 5; j++) { System.out.println("Thread Y with j = "+ j); } Organisation.out.println("Exiting Thread Y ..."); } } form ThreadZ implements Runnable{ public void run( ) { for(int thou = 1; one thousand <= 5; thousand++) { Organization.out.println("Thread Z with m = "+ k); } System.out.println("Exiting Thread Z ..."); } } form MultiThreadRunnable{ public static void main(String args[]) { ThreadX ten = new ThreadZ(); Thread t1 = new Thread(ten); ThreadY y = new ThreadY(); Thread t2 = new Thread(y); ThreadZ z = new ThreadZ(); Thread t3 = new Thread(z); t1.start(); t2.showtime(); t3.commencement(); Arrangement.out.println("... Multithreading is over "); } }
Do vi.3 /* Utilize of yield(), terminate() and sleep() methods */ class ClassA extends Thread{ public void run() { System.out.println("Starting time Thread A ...."); for(int i = ane; i <= 5; i++) { if (i==1) yield(); System.out.println("From Thread A: i = "+ i); } System.out.println("... Exit Thread A"); } } class ClassB extends Thread{ public void run() { Organisation.out.println("First Thread B ...."); for(int j = 1; j <= five; j++) { Organization.out.println("From Thread B: j = "+ j); if (j==two) stop(); } System.out.println("... Exit Thread B"); } } course ClassC extends Thread{ public void run() { System.out.println("Get-go Thread C ...."); for(int yard = 1; grand <= 5; k++) { Organisation.out.println("From Thread B: j = "+ grand); if (yard==iii){ endeavor{ slumber(g); }grab(Exception e){} } } System.out.println("... Exit Thread C"); } } form ThreadControl{ public static void principal (String args[]) { ThreadA t1 = new ThreadA(); ThreadB t2 = new ThreadB(); ThreadC t3 = new ThreadC(); t1.outset(); t2.get-go(); t3.kickoff(); Organization.out.println("... Finish of executuion "); } }
Practice half-dozen.4 /* Use of suspend() and resume() methods */ class Thread1 extends Thread { public void run( ) { Arrangement.out.println (" First thread starts running" ); sleep(10000); System.out.println (" Showtime thread finishes running" ); } } form Thread2 extends Thread { public void run( ) { Arrangement.out.println ( "Second thread starts running"); System.out.println ( "Second thread is suspended itself "); suspend( ); System.out.println (" Second thread runs again" )); } } grade AnotherThreadControl { public static void primary (Cord, args[ ] ) { Thread1 fist = new Thread1( ); // It is a newborn thread i.due east. in Newborn land Thread2 2d= new Thread2( ); // another new built-in thread get-go.start( ); // first is scheduled for running second.offset( ); // second is scheduled for running Organization.out.println("Revive the second thread" ); // If it is suspended 2nd.resume( ); System.out.println ("2d thread went for 10 seconds sleep " ); Second.sleep (10000); System.out.println ("Wake up 2nd thread and finishes running" ); System.out.println ( " Demonstration is finished "); } }
Practise 6.5 /* Setting priority to threads */ class ClassA extends Thread{ public void run() { Arrangement.out.println("Start Thread A ...."); for(int i = ane; i <= 5; i++) { Organisation.out.println("From Thread A: i = "+ i); } System.out.println("... Leave Thread A"); } } grade ClassB extends Thread{ public void run() { System.out.println("Kickoff Thread B ...."); for(int j = one; j <= five; j++) { System.out.println("From Thread B: j = "+ j); } Arrangement.out.println("... Go out Thread B"); } } grade ClassC extends Thread{ public void run() { System.out.println("Beginning Thread C ...."); for(int thousand = 1; k <= 5; k++) { Organisation.out.println("From Thread B: j = "+ j); } System.out.println("... Get out Thread C"); } } grade ThreadPriorityTest{ public static void main (String args[]) { TheadA t1 = new ThreadA(); TheadB t2 = new ThreadB(); TheadC t3 = new Thread3(); t3.setPriority(Thread.MAX_PRIORITY); t2.setPriority(Thread.getPriority() + ane); t1.setPriority(Thread.MIN_PRIORITY); t1.start(); t2.start(); t3.starting time(); System.out.println("... Terminate of executuion "); } }
Practice 6.half-dozen /* Following Java awarding create a listing of numbers and then sort in ascending guild likewise as in descending social club simultaneously. */ import coffee.util.*; class Numbers { public int result[] = new int[10]; void displayListOfNos() { Organisation.out.println("Numbers stored in the assortment:"); for( int idx=0; idx<10; ++idx) { System.out.println(result[idx]); } } void fillTheArray(int aUpperLimit, int aArraySize) { if (aUpperLimit <=0) { throw new IllegalArgumentException("UpperLimit must be positive: " + aUpperLimit); } if (aArraySize <=0) { throw new IllegalArgumentException("Size of returned List must be greater than 0."); } Random generator = new Random(); for( int idx=0; idxissue[j]) { int temp = result[i]; result[i] = result[j]; consequence[j] = temp; } } } displayListOfNos(); } } grade ArrangementAscending implements Runnable { Numbers n1 ; ArrangementAscending(Numbers due north) { n1 = n; new Thread(this).get-go(); } public void run() { n1.sortAscending(); } } class ArrangementDescending implements Runnable { Numbers n2; ArrangementDescending(Numbers n) { n2 = n; new Thread(this).start(); } public void run() { n2.sortDescending(); } } form ArrangingNos { public static void main(String args[]) { Numbers n = new Numbers(); n.fillTheArray(20,10); ArrangementAscending a1 = new ArrangementAscending(n); ArrangementDescending d1 = new ArrangementDescending(due north); } }
Do vi.seven /* The following Java awarding shows how the transactions in a bank can be carried out concurrently. */ grade Account { public int residual; public int accountNo; void displayBalance() { System.out.println("Account No:" + accountNo + "Balance: " + balance); } synchronized void deposit(int amount) { balance = balance + corporeality; System.out.println( amount + " is deposited"); displayBalance(); } synchronized void withdraw(int amount) { residue = residuum - corporeality; System.out.println( corporeality + " is withdrawn"); displayBalance(); } } class TransactionDeposit implements Runnable { int amount; Account accountX; TransactionDeposit(Account ten, int amount) { accountX = x; this.corporeality = amount; new Thread(this).start(); } public void run() { accountX.deposit(amount); } } form TransactionWithdraw implements Runnable { int amount; Account accountY; TransactionWithdraw(Account y, int corporeality) { accountY = y; this.corporeality = amount; new Thread(this).beginning(); } public void run() { accountY.withdraw(amount); } } form Transaction { public static void main(String args[]) { Account ABC = new Account(); ABC.balance = g; ABC.accountNo = 111; TransactionDeposit t1; TransactionWithdraw t2; t1 = new TransactionDeposit(ABC, 500); t2 = new TransactionWithdraw(ABC,900); } }
Assignment
| Write a Coffee program which handles Push operation and Popular functioning on stack concurrently. |
| Write a Java program which beginning generates a set of random numbers and then determines negative, positive even, positive odd numbers concurrently. |
Q&A
| What is the thread? |
| A thread is a lightweight subprocess. It is a separate path of execution because each thread runs in a different stack frame. A procedure may incorporate multiple threads. Threads share the process resources, but still, they execute independently.. |
| How to implement Threads in coffee? |
| Threads tin can be created in 2 ways i.due east. by implementing coffee.lang.Runnable interface or extending java.lang.Thread class then extending run method. Thread has its own variables and methods, it lives and dies on the heap. But a thread of execution is an individual process that has its own phone call stack. Thread are lightweight process in coffee. 1)Thread creation past implementingjava.lang.Runnableinterface. We will create object of class which implements Runnable interface : MyRunnable runnable=new MyRunnable();2) Then create Thread object by calling constructor and passing reference of Runnable interface i.eastward. runnable object : Thread thread=new Thread(runnable); |
| Does Thread implements their ain Stack, if yes how? |
| Yes, Threads have their own stack. This is very interesting question, where interviewer tends to cheque your basic noesis near how threads internally maintains their own stacks.Shown in effigy below. ![]() |
| What is multithreading? |
| Multithreading is a procedure of executing multiple threads simultaneously. Multithreading is used to obtain the multitasking. Information technology consumes less retention and gives the fast and efficient performance. Its main advantages are:
|
| What exercise yous empathise past inter-thread advice? |
| |
| How tin can you say Thread behaviour is unpredictable? |
| Thread behaviour is unpredictable because execution of Threads depends on Thread scheduler, thread scheduler may accept different implementation on different platforms similar windows, unix etc. Aforementioned threading program may produce dissimilar output in subsequent executions fifty-fifty on same platform. To achieve nosotros are going to create 2 threads on same Runnable Object, create for loop in run() method and start both threads. There is no surety that which threads will complete first, both threads will enter anonymously in for loop. |
| How can yous ensure all threads that started from main must end in order in which they started and also chief should finish in last? |
| Nosotros can use join() methodto ensure all threads that started from primary must end in order in which they started and also primary should terminate in last.In other words waits for this thread to die. Calling join() method internally calls bring together(0). |
| What is difference between starting thread with run() and outset() method? |
| When you phone call outset() method, main thread internally calls run() method to start newly created Thread, so run() method is ultimately called by newly created thread. When you call run() method main thread rather than starting run() method with newly thread it start run() method by itself. |
| What is significance of using Volatile keyword? |
| Java allows threads to admission shared variables. As a rule, to ensure that shared variables are consistently updated, a thread should ensure that it has exclusive utilise of such variables by obtaining a lock that enforces mutual exclusion for those shared variables. If a field is declared volatile, in that case the Java retentiveness model ensures that all threads see a consistent value for the variable. Note:volatile is only a keyword, tin can be used simply with variables. |
| What is race condition in multithreading and how can nosotros solve it? |
| When more than than ane thread effort to access aforementioned resource without synchronization causes race status. So we tin solve race condition by using either synchronized cake or synchronized method. When no two threads can access aforementioned resources at a time phenomenon is also called equally mutual exclusion. |
| When should nosotros interrupt a thread? |
| We should interrupt a thread when we want to break out the sleep or expect state of a thread. We tin interrupt a thread by calling the interrupt() throwing the InterruptedException. |
| What is the purpose of the Synchronized block? |
| The Synchronized block tin can be used to perform synchronization on any specific resource of the method. Only one thread at a time tin execute on a particular resource, and all other threads which effort to enter the synchronized block are blocked.
|
| What is the difference between notify() and notifyAll()? |
| The notify() is used to unblock 1 waiting thread whereas notifyAll() method is used to unblock all the threads in waiting state. |
| How to detect a deadlock status? How tin can it be avoided? |
| We tin can notice the deadlock condition by running the code on cmd and collecting the Thread Dump, and if whatever deadlock is nowadays in the code, then a message volition appear on cmd. Ways to avoid the deadlock status in Java:
|
| Difference between look() and sleep(). |
| called from synchronized block :look() method is always called from synchronized block i.east. wait() method needs to lock object monitor before object on which it is called. But sleep() method can be called from outside synchronized block i.due east. sleep() method doesn�t need any object monitor. IllegalMonitorStateException : if expect() method is called without acquiring object lock than IllegalMonitorStateException is thrown at runtime, but slumber() methodnever throws such exception. Belongs to which class: look() method belongs to java.lang.Object class but slumber() method belongs to java.lang.Thread class. Called on object or thread: wait() method is called on objects but sleep() method is called on Threads not objects. Thread state: when look() method is called on object, thread that holded object�s monitor goes from running to waiting land and can return to runnable state only when notify() or notifyAll()method is called on that object. And later thread scheduler schedules that thread to go from from runnable to running country. when sleep() is chosen on thread it goes from running to waiting land and tin can return to runnable state when sleep time is up. |
| Similarity between yield() and sleep(). |
|
|
| What are daemon threads? |
| Daemon threads are low priority threads which runs intermittently in background for doing garbage drove. Few salient features of daemon() threads>
|
| Can a constructor be synchronized? |
| No, constructor cannot be synchronized. Because constructor is used for instantiating object, when we are in constructor object is under creation. And so, until object is not instantiated it does not demand whatsoever synchronization. COMPILATION ERROR = Illegal modifier for the constructor in type ConstructorSynchronizeTest; merely public, protected & individual are permitted we can use synchronized cake within constructor. |
quisenberrywhimew.blogspot.com
Source: https://cse.iitkgp.ac.in/~dsamanta/java/ch6.htm
0 Response to "How Do You Loop the Same Thread Over and Over Again in Java"
Post a Comment